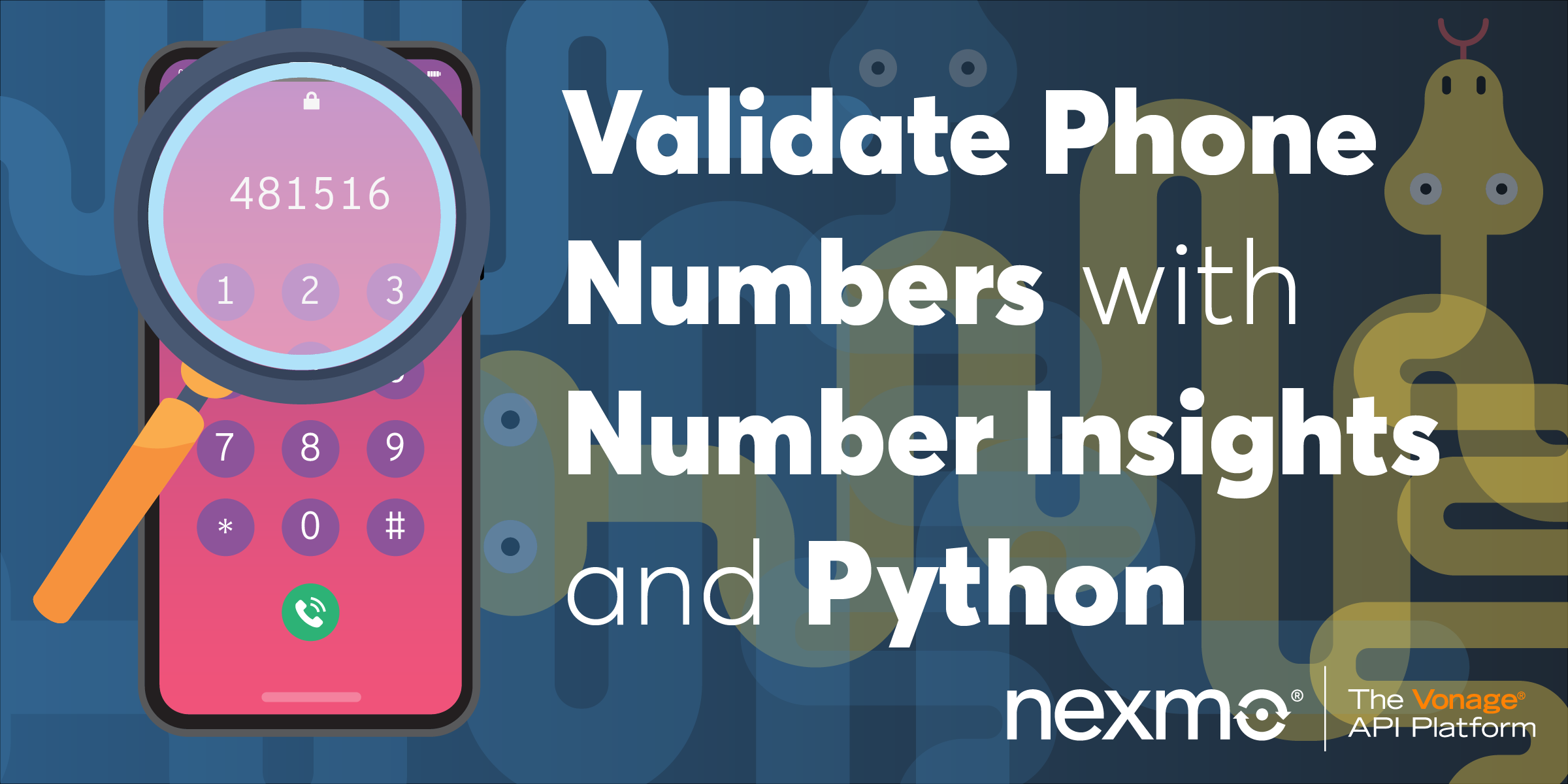
Share:
Aaron was a developer advocate at Nexmo. A seasoned software engineer and wannabe digital artist Aaron is frequently found creating things with code, or electronics; sometimes both. You can customarily tell when he's working on something new by the smell of burning components in the air.
Validate Telephone Numbers with Nexmo Number Insights and Python
Time to read: 4 minutes
Phone number verification can do a lot more than ensuring that a telephone number is formatted correctly; it can help protect you and your customers from fraud, ensure your customers are reachable and offer a better overall customer experience.
Nexmo's Number Insight API has several different tiers of information based upon the level of detail you require. I'll go through each of these in more detail below, but in summary, they are:
Basic API, used to identify which number the country is from and to ensure that it is correctly formatted. This tier is free to use.
Standard API, determine the type of number; landline, mobile (cellular), or virtual as well as the service provider.
Advanced API, our most detailed level and the most useful for discerning risk. This API provides information on number porting, roaming, validity, and reachability (not available in the US), as well as caller name and type. This Caller ID Name (CNAM) is only available for US numbers.
Prerequisites
The Nexmo Server SDK for Python. You can download this from GitHub or install from PyPI.
Vonage API Account To complete this tutorial, you will need a Vonage API account. If you don’t have one already, you can sign up today and start building with free credit. Once you have an account, you can find your API Key and API Secret at the top of the Vonage API Dashboard.
Optional Requirements
I've published the source code for the examples below on GitHub; it has a few additional requirements.
Set Up
Each of the APIs uses the Nexmo Python client, so first, we instantiate an instance of the client using our Nexmo API key and secret, which you can find on your Nexmo dashboard.
self.client = nexmo.Client(
key=os.environ["NEXMO_API_KEY"], secret=os.environ["NEXMO_API_SECRET"]
)
Basic API
Let's start with the free tier.
response = cls.client.get_basic_number_insight(number=cls.number)
Here you can see a successful response; the script is converting the object returned by the Nexmo client to a JSON string before printing to the Terminal. To make the rest of the examples more readable, I use jq to format the output.
In the example above you can see that the API takes an E.164 formatted number and returns both the international and national formatting for the number, this is useful when displaying telephone numbers in a human-readable format to your users.
Notes About the Demos
It's worth noting that while I have created a CLI to demo the usage of Number Insight, it's not a requirement to use the API. You can use the Nexmo Server SDK for Python directly within your Python scripts; the source is MIT Licensed.
While this demo only works with number insight, if you would like to access all of Nexmo's APIs from the command line we also have the Vonage CLI. You could even combine the two.
Standard API
response = cls.client.get_standard_number_insight(number=cls.number)
As well as the information provided by the Basic API, the Standard API returns information on the number type and carrier. You can see a full feature comparison table on Nexmo Developer.
Information on number type can be useful in determining how you should contact the user. For example, if the network type is mobile
, then you may use SMS for notifications, but if the network type is landline
, then you should use the Voice API. Some services choose to block users from using virtual numbers to register by ensuring the network type is not virtual
.
The Standard tier is also the first level, which charges per API call.
Advanced API
The Advanced API contains much more information and is incredibly useful in helping to prevent fraud and for protecting your users.
There is a wealth of information provided by the Advanced API, but it is still a single function call.
response = cls.client.get_advanced_number_insight(number=cls.number, cnam=True)
You need to add cnam=True
to retrieve CNAM data for US numbers, where available.
Fraud Detection and Risk Management
One of the most valuable uses of the Number Insights API is as part of your fraud detection and risk management process. Is the number valid, is it reachable, does the carrier location match other location information you have for the user; these are all examples of questions you could use within your risk scoring process during registration.
By combining the Number Insight API with Nexmo Verify, you can also protect high-value transactions within your application. Has any of the information changed since the user registered, is the CNAM different, is the number ported, has the carrier changed, is the number roaming but the user's location remains the same; again all questions which can inform your risk scores.
Location Checking
To make it easier to check that the location of a number matches that of the user, our Advanced API also includes optional IP matching. If you provide the user's IP address, we attempt to determine the country the IP address originates from and return either an ip_match_level
of country
if they match or mismatch
if they don't.
response = cls.client.get_advanced_number_insight(number=cls.number, ip=ip)
Summary
A handy way to remember which Number Insight level to use:
Basic, what country is the number from and how should it look
Standard, what type of number is it and who provided it
Advanced, does this number raise any red flags which might indicate a risk to my business or my users
Share:
Aaron was a developer advocate at Nexmo. A seasoned software engineer and wannabe digital artist Aaron is frequently found creating things with code, or electronics; sometimes both. You can customarily tell when he's working on something new by the smell of burning components in the air.