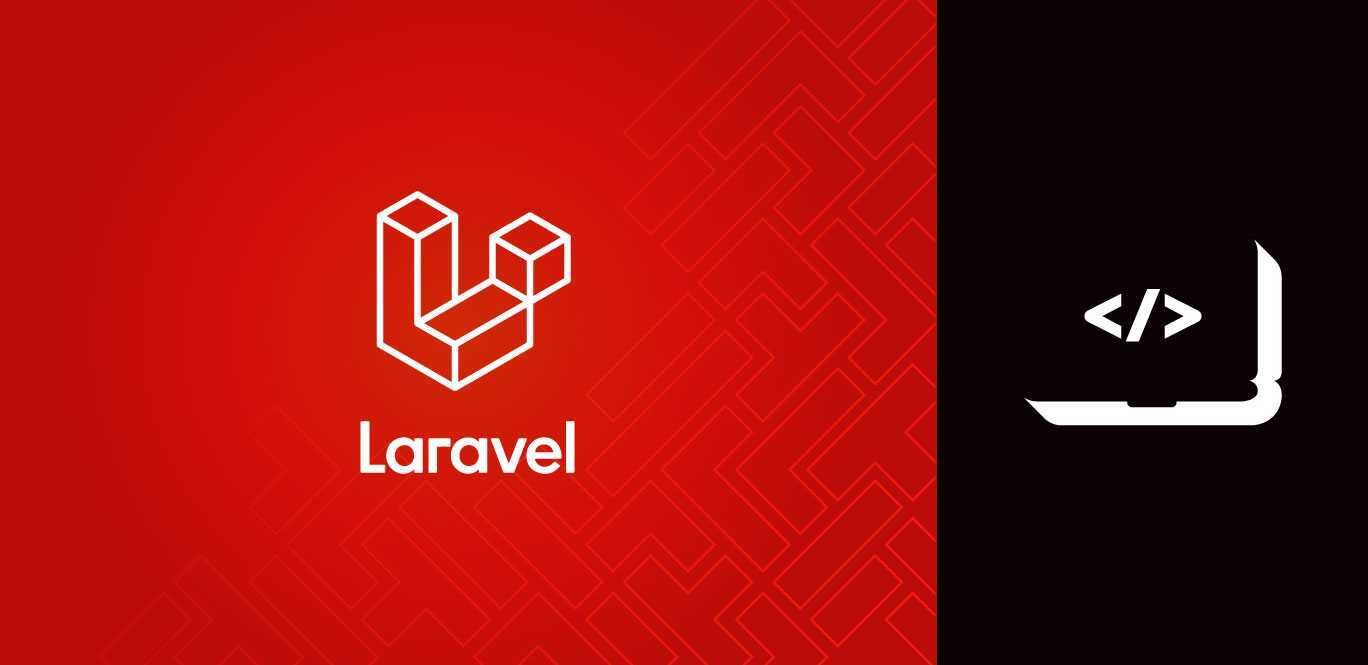
How to Use PHP Environment Variables in Laravel
Environment variables are essential in Laravel for managing configuration settings across different environments (local, staging, and production). Laravel stores these settings in a .env
file, keeping sensitive data out of version control. This ensures flexibility and security when deploying applications.
In this guide, we'll show you how to configure and use environment variables in a development environment in this case, because production and staging environments have .env
files that should be managed with a managed hosting platform such as Platform.sh, Laravel Forge, or Laravel Cloud.
Setting Up Environment Variables
Creating and Using the .env
File
Laravel includes a .env.example
file by default. To start using environment variables, create a new .env
file by copying across the example file:
Then, open the .env
file and define your application settings:
APP_NAME=VonageVerifyApp
APP_ENV=local
APP_DEBUG=true
APP_URL=http://localhost
VONAGE_API_KEY=your_api_key
VONAGE_API_SECRET=your_api_secret
After modifying the .env
file, clear the configuration cache to apply the changes:
Accessing Environment Variables in Laravel
Using the env()
Helper
Laravel provides an env()
helper to retrieve environment variables:
$apiKey = env('VONAGE_API_KEY');
Important: Use env()
only in configuration files. To keep application logic clean and maintainable, retrieve settings via Laravel's config()
helper instead.
Using Laravel Configuration Files
Laravel's configuration files (found in the config/
directory) are the best way to manage environment settings. Laravel's config service container automatically reads each file within the config directory. So, to add your Vonage key and API secret, create a new file in config and name it vonage.php
. This should return an array with your key and API secret.
For instance, in config/services.php
, you can reference environment variables for the Vonage Verify API:
'vonage' => [
'api_key' => env('VONAGE_API_KEY'),
'api_secret' => env('VONAGE_API_SECRET'),
],
To retrieve these values in your application, use:
$apiKey = config('vonage.api_key');
$apiSecret = config('vonage.api_secret');
Using Environment Variables in the Verify API
With the environment variables properly set up, you can now integrate the Vonage Verify API into your Laravel application. We'll show you how to do this manually, but when building your app, you'll probably want to write a service that automatically boots your app in the service container. You can learn more about how to do this in the Laravel documentation.
Example: Sending a Verification Request
use Vonage\Client;
use Vonage\Client\Credentials\Basic;
use Vonage\Verify2\Request\SMSRequest;
$apiKey = config('vonage.api_key');
$apiSecret = config('vonage.api_secret');
$credentials = new Basic($apiKey, $apiSecret);
$client = new Client($credentials);
$verification = new SmsRequest('+14155550123', 'acme-company');
$response = $client->verify()->start($verification);
echo "Request ID: " . $response->getRequestId();
Example: Checking a Verification Code
$requestId = 'VERIFY_REQUEST_ID';
$code = 'VONAGE_VERIFY_CODE; // Code entered by the user
try {
$response = $client->verify2()->check($requestId, $code);
echo $response;} catch ($exception) {
echo $exception->getMessage();
}
Configuring Environment-Specific Settings
Laravel allows you to customize settings for different environments. For example, in config/logging.php
, you can set different log levels based on the environment. The second argument into the env() helper function is the default, so make sure you know where you are setting this:
'log_level' => env('LOG_LEVEL', 'debug'),
Define the log level in your .env
files:
LOG_LEVEL=debug # Local Development
LOG_LEVEL=error # Production
This approach lets you modify application behavior without changing the code.
Improving Performance with Configuration Caching
For production environments, cache your configuration settings for improved performance:
After running this command, Laravel stops reading from the .env
file and uses the cached configuration instead. If you make changes to .env
, clear the cache before applying them:
Security Best Practices
To keep sensitive data safe, follow these best practices:
Never commit your
.env
file—add it to.gitignore
.Use separate environment files for local, staging, and production.
Never hardcode API keys or secrets in your application code.
Use
config()
instead ofenv()
in application logic to avoid issues with configuration caching.Consider secret management tools like AWS Secrets Manager, HashiCorp Vault, or Docker/Kubernetes environment management for production security.
Troubleshooting Environment Variables
If your environment variables aren't loading correctly, try to clear and re-cache:
Conclusion
And that’s it! Using environment variables in Laravel helps manage configuration settings efficiently while keeping sensitive data secure.
Got any questions or comments? Join our thriving Developer Community on Slack, follow us on X (formerly Twitter), or subscribe to our Developer Newsletter. Stay connected, share your progress, and keep up with the latest developer news, tips, and events!