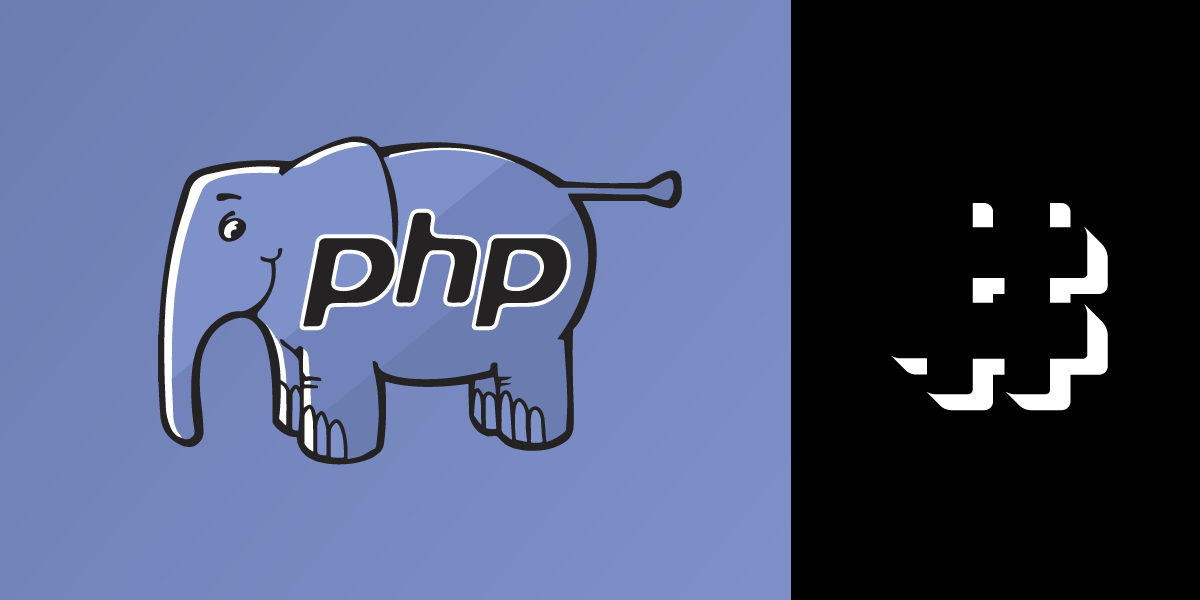
Share:
)
Lorna is a software engineer with an incurable blogging habit. She tries to tame words and code in equal measure.
How to Use The Number Insight API with PHP
Time to read: 5 minutes
In this post I'll show you an example application I made recently that uses the Nexmo Number Insight API to validate and gain information about any phone number. You could either use my project as a starting point (the code is on GitHub), or adapt the examples here to use in your own applications. Number Insights API is ideal for checking that a phone number is valid, current, and in a particular geography so it's commonly used to verify contact details at signup time.
Before You Begin
Vonage API Account
To complete this tutorial, you will need a Vonage API account. If you don’t have one already, you can sign up today and start building with free credit. Once you have an account, you can find your API Key and API Secret at the top of the Vonage API Dashboard.
This tutorial also uses a virtual phone number. To purchase one, go to Numbers > Buy Numbers and search for one that meets your needs.
This example uses PHP and a few dependencies installed via Composer.
You will want to get the code from the GitHub repository - clone or download this to your development machine.
About Number Insights API
The Number Insights API gives information about a given phone number, and is available in various levels of service with corresponding pricing levels.
The Basic level is free and is very useful to check that a number exists and is valid. Using a tool like this is great practice if you expect users to supply valid contact details because it enables you to check that this is indeed the case.
The Standard level gives everything from Basic plus information about the type of number and also the carrier it uses. With Advanced, all the above is included along with roaming and reachability information.
The Advanced Number Insight API is also available as an asynchronous call. You can find examples of this on the developer portal.
There is a good feature comparison table available in the developer documentation.
Set Up The Application
To begin, change into the directory where you put the project code. Use Composer to install the dependencies by running this command:
Copy config.php.sample
to config.php
and edit to add your Nexmo API key and secret (these can be found in your dashboard).
Finally, change into the public/
directory and start the webserver:
If you visit http://localhost:8080/ in your browser, you will see a form to input the number you are interested in and what level of insight to go for.
Screenshot of number insights form
Number Insights API with PHP
One of the dependencies of the project is nexmo/client
, and it makes it very easy for us to use the API from any PHP application. You've seen that I'm using SlimPHP today but the PHP library can be used in any framework or other project.
Take a look around the project structure, most of the PHP action is in public/index.php
. The top level route simply loads a page template (look in templates/main.php
to see it) so that the user can see the form. The form submits to /insight
and this route is where most of the activity occurs.
$params = $request->getParsedBody();
$basic = new \Nexmo\Client\Credentials\Basic(
$this->config['api_key'],
$this->config['api_secret']
);
$client = new \Nexmo\Client($basic);
// choose the correct insight type
switch($params['insight']) {
case "standard":
$insight = $client->insights()->standard($params['number']);
break;
case "advanced":
$insight = $client->insights()->advanced($params['number']);
break;
default:
$insight = $client->insights()->basic($params['number']);
break;
}
First we grab those form parameters into $params
(this helper function is the only Slim-specific bit!), then we instantiate the \Nexmo\Client
object and supply our API key and secret to it.
Next, there's a switch statement so that the correct Number Insights API endpoint gets called, with "basic" as the default type - because we never trust user input! It's a form, they could send us anything.
The response gets stored in $insight
and the action passes it through to the template for display. The $insight
value is an object, but its data fields are accessible via array notation, for example $insight['status']
or $insight['country_code'
. In the template, the fields are displayed in a tabular layout, showing the fields that were returned for this level of Number Insight, as shown below:
Screenshot of the number insights output
There is also handling for an error outcome: if status
is non-zero, that indicates an error and the status code and message are passed through to the template and displayed there above the form. You can test this by entering an invalid phone number.
Further Reading
Here are some places you might like to go next:
The API Reference docs are probably a good place to start!
Code Snippets are available on the developer portal in other programming languages.
For one-off number lookups, you could use Number Insights API from the CLI.
There's a tutorial on Fraud Scoring and Phone Number Verification (warning, contains Ruby).