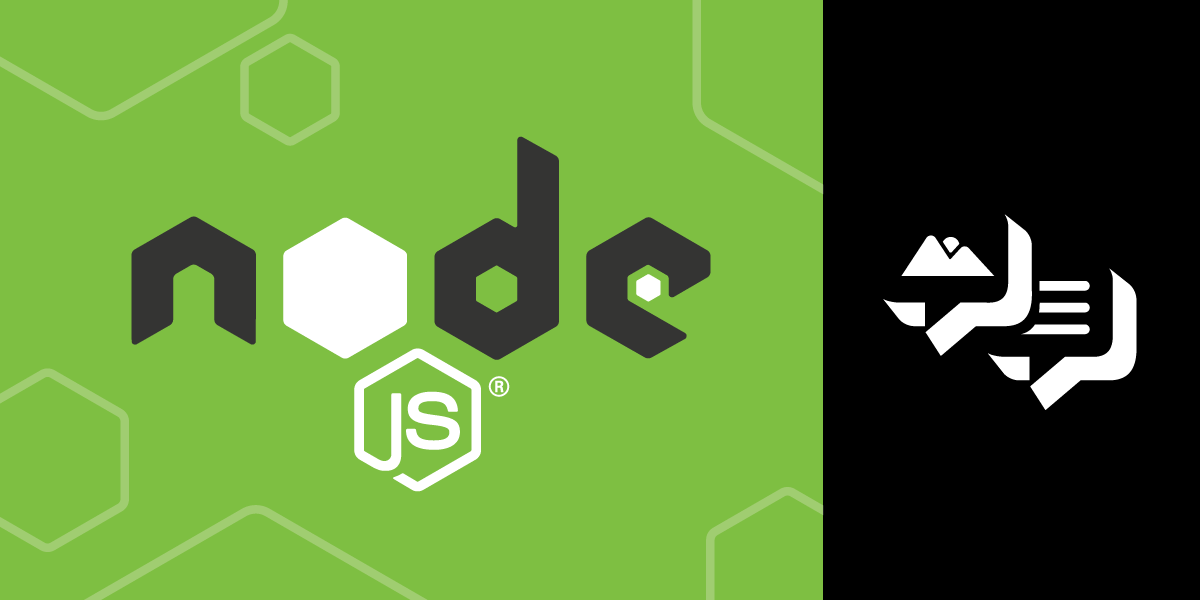
How to Send MMS Messages with Node.js
The Vonage Messages API now allows you to send MMS (Multimedia Messaging Service) from US 10DLC, TFN, and Shortcode numbers you have in your dashboard to other numbers within the United States.
In this article, you will learn how to send an MMS using Node.js using the official vonage-node-sdk client library.
Prerequisites
Before you start, make sure you have the following:
A basic understanding of JavaScript & Node.js.
Node.js installed on your machine.
A fresh US SMS & MMS capable number. You can reference our Numbers Guide for details on how to buy a number if you don't have one.
Note: MMS messaging only works for A2P (Application to Person) use cases. The Vonage Messages API cannot be used to send MMS messages from one virtual number to another virtual number.
Vonage API Account
To complete this tutorial, you will need a Vonage API account. If you don’t have one already, you can sign up today and start building with free credit. Once you have an account, you can find your API Key and API Secret at the top of the Vonage API Dashboard.
This tutorial also uses a virtual phone number. To purchase one, go to Numbers > Buy Numbers and search for one that meets your needs.
Getting Started
Before we begin, let's get set up for success by taking care of a few things inside the Vonage dashboard.
Let's start by creating a new Messages Application. To do this, head to your admin dashboard's Application section of your admin dashboard and click on Create a new application.
Your applications page
Next, you will need to fill out the form with your application name and the required webhook URLs.
Every Messages application requires you to specify a Status URL
and an Inbound URL
. In larger, production-ready applications, you would have these pointing to a URL on your own server.
There is no need for that in this tutorial; you'll need a URL that can respond with a 200 OK
status. You can use a service such as MockBin or ngrok to provide what you need.
To use MockBin in this example, you can:
Go to the MockBin website and click on the green button that says "Create Bin".
In the Bin Builder, leave the default pre-filled information that says Status Code 200 OK, and at the bottom, click on Create Bin.
That will generate an URL that looks like https://mockbin.org/bin/2f27d0d5-6f2e-4a39-8dd5-918c4848a4c7/view. Copy the URL up to before /view and paste it onto the Vonage Dashboard under the Messages Capability in both Inbound URL and Status URL as per the image below.
Message capability with added URLs
In order to authenticate API requests alongside your API key and API Secret, you will also need a public/private key pair, which can be automatically generated for you by clicking the Generate public/private key pair link as per the image below.
Generate Public and Private Key
This will set a public key in the form field and also download a private.key
file to your machine. You will need to put this file in the directory we will create shortly.
Finish up by clicking Create a new application. You will then be asked to select a number to use with this application by clicking the Link button next to your number of choice. If you do not have any numbers on your dashboard, you can purchase them there or via the command line using the Vonage CLI.
Note down the Application ID. It will join your API key, API Secret and Private Key in the code we will work on next.
Sending MMS Using Node.js
Now that the admin is complete, let's create a Node.js script that will use the Vonage Server SDK for Node.js to send an MMS to a pre-set number when run.
Start by creating a new folder (and if you haven't already done so, put the private.key
file generated earlier in it.
From the terminal, run the following commands to set up a new Node.js application and install the vonage-node
client library inside a new folder for your project.
npm init -y
npm install -g @vonage/cli
Then create a new file called send-mms.js
, and open it inside your editor.
Initialise a new Vonage instance:
const { Messages, MMSImage } = require("@vonage/messages");
const messageClient = new Messages({
apiKey: VONAGE_API_KEY, // Found in your Vonage Dashboard
apiSecret: VONAGE_API_SECRET, // Found in your Vonage Dashboard
applicationId: VONAGE_APPLICATION_ID, // Generated earlier
privateKey: VONAGE_APPLICATION_PRIVATE_KEY_PATH // Generated earlier it could be e.g. ./private.key
});
Finally, add the code to send the MMS message using the Messages API:
const image = new MMSImage({
image: {
url: "https://placekitten.com/200/30",
caption: "placeholder image"
},
to: "TO_NUMBER", // Add the phone number sending the message
from: "FROM_NUMBER", // Add the phone number receiving the nmessage
});
messageClient
.send(image)
.then((resp) => console.log(resp))
.catch((err) => console.error(error));
The above code sends a single message (a picture of a cat) to any number you add to the first object. If, for any reason, there is a failure in sending the message, the issues will be logged to the console; otherwise, if everything works as it should, then the messageUUID
will be logged instead.
Notes:
'https://placekitten.com/200/300' is a placeholder image you can use.
The value for
url
has to be a publicly accessible URL that resolves to a file.The file types supported are
.jpg
,.jpeg
, and.png
.
With that, head to your terminal of choice and run:
node send-mms.js
Where To Go From Here
Sometimes, for any number of reasons, an MMS message may not be delivered to a recipient so, as a next step from this project, you could experiment with our Dispatch API and build a failover mechanism that checks whether the MMS has been delivered, and if not, your app would instead send a standard SMS message with a link to the image you wanted to include.
Let Us Know What You're Building With MMS
Join the conversation on our Vonage Community Slack or send us a message on X, formerly known as Twitter.