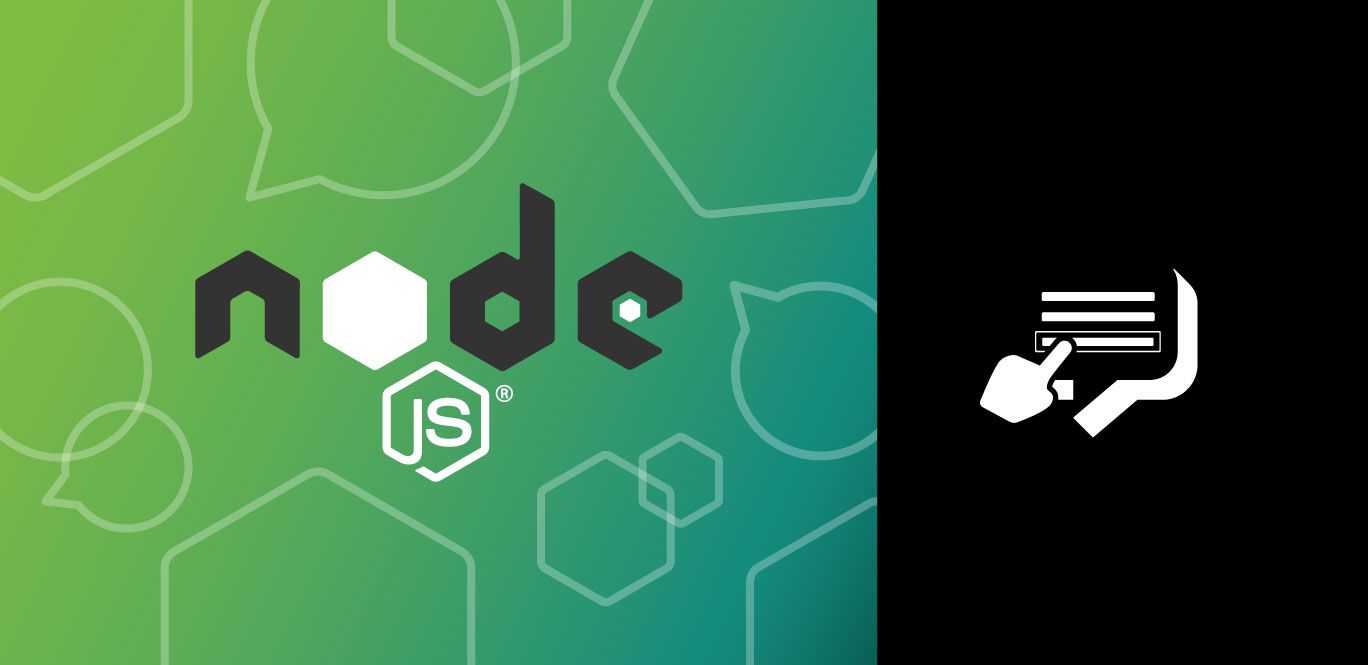
How to Send and Receive RCS Suggested Replies with Node.js
Rich Communication Services (RCS) is transforming the way businesses engage with customers. As the next evolution of SMS, RCS offers a richer, more interactive experience with features like images, videos, file sharing, and suggested replies, natively inside a user's default messaging app. Check out all the cool RCS capabilities.
With RCS support rolling out around the world, for both Android and iOS, now is the perfect time to start exploring this technology. In this tutorial, you'll learn how to send RCS suggested replies using the Vonage Messages API in a Node.js application, helping you create more dynamic and engaging conversations with your users.
TL;DR: Find the complete working code on GitHub.
What Are RCS Suggested Replies?
RCS Suggested Replies enhance user interactions by providing predefined response options within messages. When a user selects a suggested reply, your application receives a structured response, allowing for streamlined and interactive conversations.
RCS message sent using the Vonage Messages API, prompting the user to select a preferred appointment time with suggested reply buttons.
Prerequisites
Before you begin, ensure you have the following:
Node.js installed on your machine.
ngrok installed for exposing your local server to the internet.
A Vonage API account.
A registered RCS Business Messaging (RBM) Agent.
A phone with RCS capabilities for testing.
Vonage API Account
To complete this tutorial, you will need a Vonage API account. If you don’t have one already, you can sign up today and start building with free credit. Once you have an account, you can find your API Key and API Secret at the top of the Vonage API Dashboard.
How to Contact Your Vonage Account Manager
In order to send and receive RCS capabilities in your Vonage application, you will need to have a registered Rich Business Messaging (RBM) agent and a phone with RCS capabilities.
Currently, RCS Messaging through Vonage is only available for managed accounts. You will need to contact your account manager to request Developer Mode activation for your RBM agent. Developer Mode allows you to test RCS messaging to allow-listed numbers before completing the Agent Verification process and launching in production.
Please contact our sales team if you do not have a managed account.
>> Understand the difference between RCS and RBM.
How to Set Up Your Node.js Project
This tutorial will assume you have a working understanding of JavaScript and Node, sticking to the commands required without too much deeper explanation.
Initialize the Project
Create a new directory and initialize a Node.js project:
mkdir rcs-suggested-replies-node
cd rcs-suggested-replies-node
npm init -y
Install Project Dependencies
Install the necessary node packages with Node Package Manager:
npm install express dotenv @vonage/server-sdk
express
: Creates the web server.dotenv
: Loads environment variables securely.@vonage/server-sdk
: The Vonage Node SDK for interacting with the Messages API
Create Your Project Files
Create the main application file and environment configuration file:
touch index.js .env
How to Configure Environment Variables
In the .env file, add your Vonage credentials and configuration:
VONAGE_APPLICATION_ID=your_application_id
VONAGE_PRIVATE_KEY_PATH=./private.key
RCS_SENDER_ID=your_rbm_agent_id
PORT=3000
VONAGE_API_SIGNATURE_SECRET=your_api_secret
VONAGE_APPLICATION_ID
: Your Vonage application ID.VONAGE_PRIVATE_KEY
: Your private key file.RCS_SENDER_ID
: Your RBM SenderID (the Name of the Brand). The SenderID requires special formatting, such as not having any spaces. Check with your account manager if you’re unsure.PORT
: Port number for the Express server.VONAGE_API_SIGNATURE_SECRET
=your_api_secret
You will obtain your Vonage Application ID and private.key file below, in the “How to Create and Configure Your Vonage Application” section.
How to Send RCS Suggested Replies
In index.js
, set up the Express server and implement the functionality to send RCS messages with suggested replies.
Load Dependencies and Initialize the Vonage Client
const express = require('express');
const fs = require('fs');
const dotenv = require('dotenv');
const { Vonage } = require('@vonage/server-sdk');
const { verifySignature } = require('@vonage/jwt');
dotenv.config();
const app = express();
app.use(express.json());
const PORT = process.env.PORT || 3000;
const VONAGE_API_SIGNATURE_SECRET = process.env.VONAGE_API_SIGNATURE_SECRET;
const privateKey = fs.readFileSync(process.env.VONAGE_PRIVATE_KEY);
const vonage = new Vonage({
applicationId: process.env.VONAGE_APPLICATION_ID,
privateKey: privateKey
});
Define an Express Endpoint to Send Suggested Replies
app.post('/send-rcs', async (req, res) => {
const toNumber = req.body.to;
const message = {
to: toNumber,
from: process.env.RCS_SENDER_ID,
channel: 'rcs',
message_type: 'custom',
custom: {
contentMessage: {
text: "What time works best for your appointment?",
suggestions: [
{
reply: {
text: "9am",
postbackData: "time_9am"
}
},
{
reply: {
text: "11am",
postbackData: "time_11am"
}
},
{
reply: {
text: "2pm",
postbackData: "time_2pm"
}
}
]
}
}
};
try {
const response = await vonage.messages.send(message);
console.log('Message sent: ', response);
res.status(200).json({ message: 'RCS message sent successfully.' });
} catch (error) {
console.error('Error sending message:', error);
res.status(500).json({ error: 'Failed to send RCS message.' });
}
});
How to Receive RCS Replies via Webhooks
Set up an endpoint to handle incoming RCS replies.
app.post('/inbound_rcs', async (req, res) => {
const token = request.headers.authorization.split(' ')[1];
if (!verifySignature(token, VONAGE_API_SIGNATURE_SECRET)) {
res.status(401).end();
return;
}
const inboundMessage = req.body;
if (inboundMessage.channel === 'rcs' && inboundMessage.message_type === 'reply') {
const userSelection = inboundMessage.reply.title;
const userNumber = inboundMessage.from;
console.log(`User ${userNumber} selected: ${userSelection}`);
// Optionally, send a confirmation message back to the user
const confirmationMessage = {
to: userNumber,
from: process.env.RCS_SENDER_ID,
channel: 'rcs',
message_type: 'text',
text: `${userSelection} is a great choice!`
};
try {
const response = await vonage.messages.send(confirmationMessage);
console.log('Confirmation sent:', response);
} catch (error) {
console.error('Error sending confirmation:', error);
};
}
res.status(200).end();
});
Notice that all you do with the inbound message is log it to the console. Here is where you might want to save the message to your database or create some custom logic. For this example, you simply confirm to the user you've received their reply.
Lastly, add your Express Server:
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
>> See the full index.js file.
Terminal output showing a Node.js server sending an RCS message with suggested replies and logging the user's selected response.
How to Expose Your Server with ngrok
To receive webhooks from Vonage, your local server must be accessible over the internet. Use ngrok to expose your server:
ngrok http 3000
Note the HTTPS URL provided by ngrok (e.g., https://your-ngrok-subdomain.ngrok.io).
You can read more about testing with ngrok in our developer portal tools.
How to Create and Configure Your Vonage Application
Now that your Node App is ready, you’ll also need to create and set up your Vonage Application. First, create your app in the Vonage Dashboard. Give the app a name and turn on the Messages capability.
A screenshot of the Vonage Developer dashboard showing the creation of a new application with authentication, privacy, and messaging capabilities settings.
In your Vonage application settings:
Set the Inbound URL to https://YOUR_NGROK_URL/inbound_rcs.
Set the Status URL to https://example.com/rcs_status.** Message statuses will be covered in a future article.
Generate a public and private key by clicking the button. Ensure to move your private.key file to the project root directory (rcs-suggested-replies-node).
Save the changes.
Then link your RCS Agent by clicking the “Link external accounts” tab:
Dashboard view showing the Vonage-Node-RCS application linked to the Vonage RoR RCS external account, with voice and message capabilities enabled.
How to Test Your Node RCS Application
Start your Express server:
node index.js
Use a tool like Postman or cURL to send a POST request to your /send-rcs endpoint with the recipient's phone number:
curl -X POST https://**YOUR_NGROK_URL***/send-rcs \
-H "Content-Type: application/json" \
-d '{
"to": "**YOUR_RCS_TEST_NUMBER"
}'
On the recipient's RCS-enabled device, the message with suggested replies should appear.
When the recipient selects a suggested reply, your /inbound_rcs endpoint will handle the response, and a confirmation message will be sent back.
RCS conversation where a user selects a time from suggested replies and receives a confirmation message, powered by the Vonage Messages API.
Conclusion
Great job! You've successfully implemented sending and receiving RCS Suggested Replies using Node.js and the Vonage Messages API. But RCS offers so much more! You can try sending high-resolution images and videos, or RCS-specific Rich Cards.The Messages API also provides message statuses, which help us understand what’s happening with our RCS messages. In a future blog post, I’ll show how to track message statuses with webhooks.
If you have any questions or suggestions for more Node or RCS content, join the conversation with us on the Vonage Community Slack or reach out on X, formerly known as Twitter. We’d love to hear what you build next!
Share:
)
Benjamin Aronov is a developer advocate at Vonage. He is a proven community builder with a background in Ruby on Rails. Benjamin enjoys the beaches of Tel Aviv which he calls home. His Tel Aviv base allows him to meet and learn from some of the world's best startup founders. Outside of tech, Benjamin loves traveling the world in search of the perfect pain au chocolat.