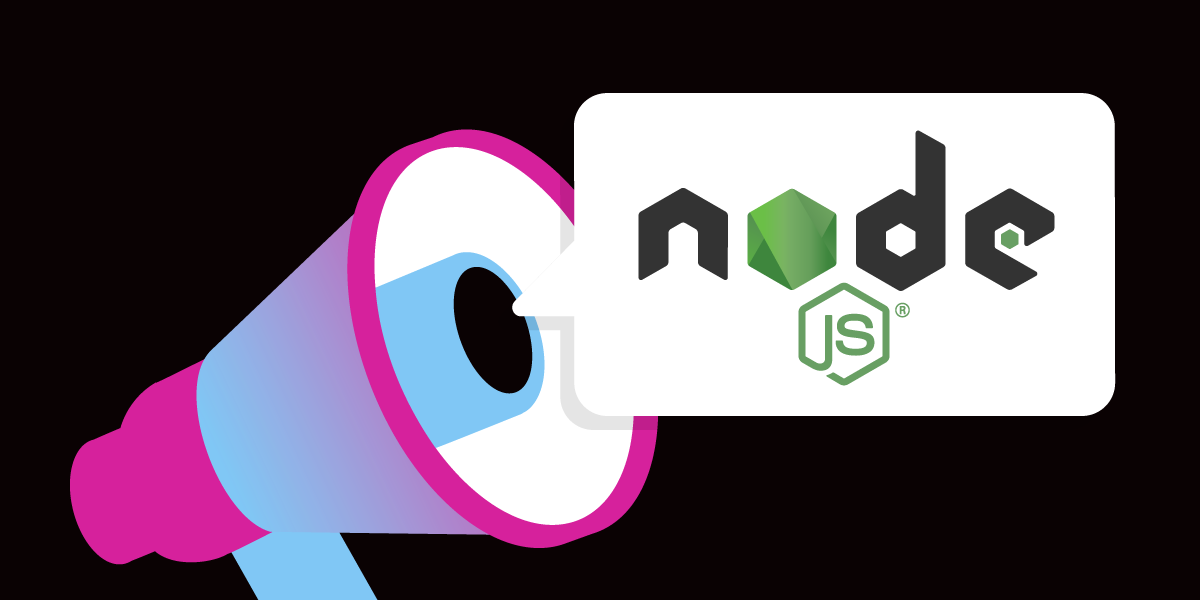
Share:
)
Long ago, in the dark ages before Google and StackOverflow, Chuck learned how to program. Those were the times when all you had to go on was the documentation or the source code itself. From humble beginnings as a Jr Full Stack developer, Chuck grew into the developer he is today, building out the tools that empower fellow developers to create amazing products. When he is not forging new tools, you can find him climbing up a mountain or on his bike.
Announcing the Vonage Node SDK v3.0.0
Time to read: 1 minute
That's right, v3 of the node SDK is now fully promised. A long-asked-for feature has been released, along with breaking out the SDK into smaller components, which will help the team release new features more quickly.
Breaking Changes
Promises
All function callbacks have been removed. In the future, you will need to use async/await
or .then/.catch
. This should make your code cleaner by avoiding callback hell. For example:
const Vonage = require('@vonage/server-sdk')
const vonage = new Vonage({
apiKey: VONAGE_API_KEY,
apiSecret: VONAGE_API_SECRET,
})
const from = VONAGE_BRAND_NAME;
const to = TO_NUMBER;
const text = 'A text message sent using the Vonage SMS API'
vonage.message.sendSms(from, to, text, (err, responseData) => {
if (err) {
console.log(err);
return;
}
if(responseData.messages[0]['status'] === "0") {
console.log("Message sent successfully.");
return;
}
console.log(`Message failed with error: ${responseData.messages[0]['error-text']}`);
})
It can now be written as:
const { Vonage } = require('@vonage/server-sdk')
const vonage = new Vonage({
apiKey: VONAGE_API_KEY,
apiSecret: VONAGE_API_SECRET,
})
const from = VONAGE_BRAND_NAME;
const to = TO_NUMBER;
const text = 'A text message sent using the Vonage SMS API';
const sendSMS = async () {
try {
const response = await vonage.sms.send({to, from, text});
console.log(response);
} catch (err) {
console.log('There was an error sending the messages.');
console.error(err);
}
};
Parameter Updates
Functions have also been updated to use parameter objects instead of function arguments. This will help keep functions with many optional parameters more manageable. We have published a migration document for each package that will describe which functions have been updated in more detail.
Migration guide
You can find migration guides for each package here:
Smaller Packages and Typescript
Version 3 uses Typescript to help with code completion in your preferred IDE. But an even bigger improvement is the use of monorepos. A monorepo breaks up the code base into smaller packages while keeping the dependancies tree intact. Now you can use specific package(s) in your application rather than including the entire suite of products. We made this change strategically to allow us to release beta features and fixes without holding up the main development branch. One example of this is the new Video API package (which in the past was part of the OpenTok SDK):
import { Auth } from '@vonage/auth';
import { Video } from '@vonage/video';
const credentials = new Auth({
apiKey: VONAGE_API_KEY,
apiSecret: VONAGE_API_SECRET,
});
const video = new Video(credentials);
const getVideoSession = async () => {
try {
const session = await video.createSession({ mediaMode: 'routed' });
console.log('Session created', session);
return session;
} catch (error) {
console.log('Failed to create session');
console.error(error);
throw error;
}
}
Now you can use a beta SDK package and keep the stable packages.
Support
Following our support document, we will continue to support version 2 for another six months bringing us to around May of 2023. We will not be adding new features, just fixes.
For more detailed information, please visit the SDK documentation or look at the code snippets. You can reach out to us on the Vonage Developer Slack and stay in the loop by following VonageDev on Twitter.
Share:
)
Long ago, in the dark ages before Google and StackOverflow, Chuck learned how to program. Those were the times when all you had to go on was the documentation or the source code itself. From humble beginnings as a Jr Full Stack developer, Chuck grew into the developer he is today, building out the tools that empower fellow developers to create amazing products. When he is not forging new tools, you can find him climbing up a mountain or on his bike.