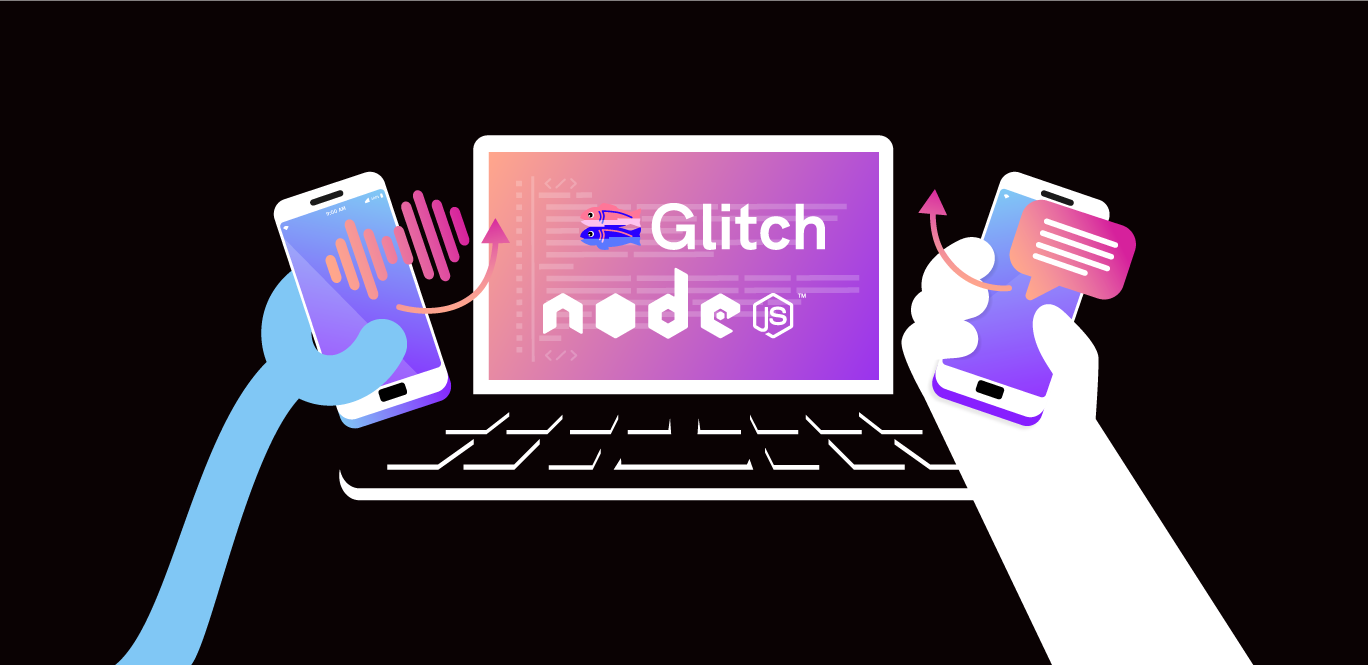
How to Make Outbound Voice & SMS with Vonage on Glitch
In this tutorial, we’ll show you how to integrate and make outbound voice calls and send outbound SMS using Vonage APIs with Glitch and Node.js. Adding outbound voice and SMS features allows your application to handle proactive interactions, such as sending notifications or making calls. This integration improves user engagement by enabling automated, real-time communication from your app.
Ready to build an even more interactive application? Let’s go!
Prerequisites
A Vonage developer account
Vonage API Account
To complete this tutorial, you will need a Vonage API account. If you don’t have one already, you can sign up today and start building with free credit. Once you have an account, you can find your API Key and API Secret at the top of the Vonage API Dashboard.
Set Up and Configure Your Project
Assuming you have already integrated a webhook for inbound voice calls and inbound SMS in your Vonage application, we’ll move on to implementing outbound features. If you need help setting up your webhook and Vonage application, you can follow our tutorial on How to Set Up Webhooks for Inbound SMS & Voice on Glitch.
To implement the application, you can apply the knowledge gained from the tutorial to set the following:
private.key
fileVonage phone number
Vonage API key and secret
Vonage application ID
Answer URL
We’ll walk you through the following steps:
Add client code for outbound voice calls and SMS
Install dependencies
Set environment variables
Test your new features
Step 1: Add Client Code for Outbound Voice Calls and SMS
Log into your Glitch account.
In your Glitch project, click on the "+" next to Files.
Name the file private.key
and click Add this File.
add fileIn your favorite text editor, open the
private.key
file you downloaded earlier. Copy the contents from your text editor and paste them into the newly created private.key
file in Glitch.
Similarly, create a new file named makecall.js
.
Add the following code to the newly created makecall.js
file:
require('dotenv').config({ path: __dirname + '/../.env' });
const { Vonage } = require('@vonage/server-sdk');
const { NCCOBuilder, Talk, OutboundCallWithNCCO } = require('@vonage/voice')
const TO_NUMBER = process.env.TO_NUMBER;
const VONAGE_NUMBER = process.env.VONAGE_NUMBER;
const VONAGE_API_KEY = process.env.VONAGE_API_KEY;
const VONAGE_API_SECRET = process.env.VONAGE_API_SECRET;
const VONAGE_APPLICATION_ID = process.env.VONAGE_APPLICATION_ID;
const VONAGE_APPLICATION_PRIVATE_KEY_PATH = __dirname + "/" + process.env.VONAGE_APPLICATION_PRIVATE_KEY_PATH;
const vonage = new Vonage({
apiKey: VONAGE_API_KEY,
apiSecret: VONAGE_API_SECRET,
applicationId: VONAGE_APPLICATION_ID,
privateKey: VONAGE_APPLICATION_PRIVATE_KEY_PATH
});
async function makeCall() {
const builder = new NCCOBuilder();
builder.addAction(new Talk('This is a text to speech call from Vonage'));
const resp = await vonage.voice.createOutboundCall(
new OutboundCallWithNCCO(
builder.build(),
{ type: 'phone', number: TO_NUMBER },
{ type: 'phone', number: VONAGE_NUMBER}
)
);
console.log(resp);
}
makeCall();
Next, create a new file named sendsms.js
.
Add the following code to the newly created sendsms.js
file:
require('dotenv').config({ path: __dirname + '/../.env' });
const VONAGE_API_KEY = process.env.VONAGE_API_KEY;
const VONAGE_API_SECRET = process.env.VONAGE_API_SECRET;
const TO_NUMBER = process.env.TO_NUMBER;
const VONAGE_NUMBER = process.env.VONAGE_NUMBER;
const { Vonage } = require('@vonage/server-sdk');
const vonage = new Vonage({
apiKey: VONAGE_API_KEY,
apiSecret: VONAGE_API_SECRET
});
const from = VONAGE_NUMBER;
const to = TO_NUMBER;
const text = 'Test message using the Vonage SMS API';
vonage.sms.send({ to, from, text })
.then(resp => { console.log('Message sent successfully'); console.log(resp); })
.catch(err => { console.log('Error sending message'); console.error(err); });
Step 2: Install dependencies
Open a terminal in your Glitch project.
Run the following command to update existing packages:
npm update
Install the required dependencies with the following command:
npm i express @fastify/express body-parser dotenv @vonage/server-sdk @vonage/voice
Run the refresh
command to apply changes:
refresh
Step 3: Set Environment Variables
Now, open the .env
file found on the left pane in Glitch.
Add the following variable names:
TO_NUMBER
VONAGE_NUMBER
VONAGE_API_KEY
VONAGE_APPLICATION_ID
VONAGE_API_SECRET
VONAGE_APPLICATION_PRIVATE_KEY_PATH
Next, fill in the following values for these variables:
TO_NUMBER
: The number to receive calls and SMS (e.g., 14567891234).VONAGE_NUMBER
: The number assigned to your Vonage Application (e.g., 14567891234).VONAGE_API_KEY
andVONAGE_API_SECRET
: From the Vonage Dashboard.VONAGE_APPLICATION_ID
: From the Vonage Application settings.VONAGE_APPLICATION_PRIVATE_KEY_PATH
: Set asprivate.key
.
Environment variables
Step 4: Test Your New Features
Test Outbound Voice Calls
Open a terminal in your Glitch project.
Run this command:
node makecall.js
Check the Vonage Dashboard logs to confirm the call was made successfully and also its details.
Outbound Call LogTest Outbound SMS
Now that you’ve added client code for outbound SMS, let’s test it!
Run this command:
node makecall.js
Check the Vonage Dashboard logs to confirm the SMS was sent successfully and also its details.
Text Log DeliveredYou'll see the same information in your terminal.
Conclusion
And that’s it! You've just integrated outbound voice calls and SMS using Vonage APIs with Glitch. By adding these communication features, you’ve expanded your application’s ability to engage users through voice and messaging. If you followed this tutorial for your own projects, we’d love to hear about it! Join the conversation on our Vonage Community Slack or send us a message on X.
Share:
)
Prashant is a member of the API Partner Sales Team at Vonage. He is based in Singapore. He drives the enablement of customers and partners to leverage Vonage CPaaS capabilities for their businesses. In his spare time, Prashant enjoys cycling the scenic island or sweating out in badminton games.