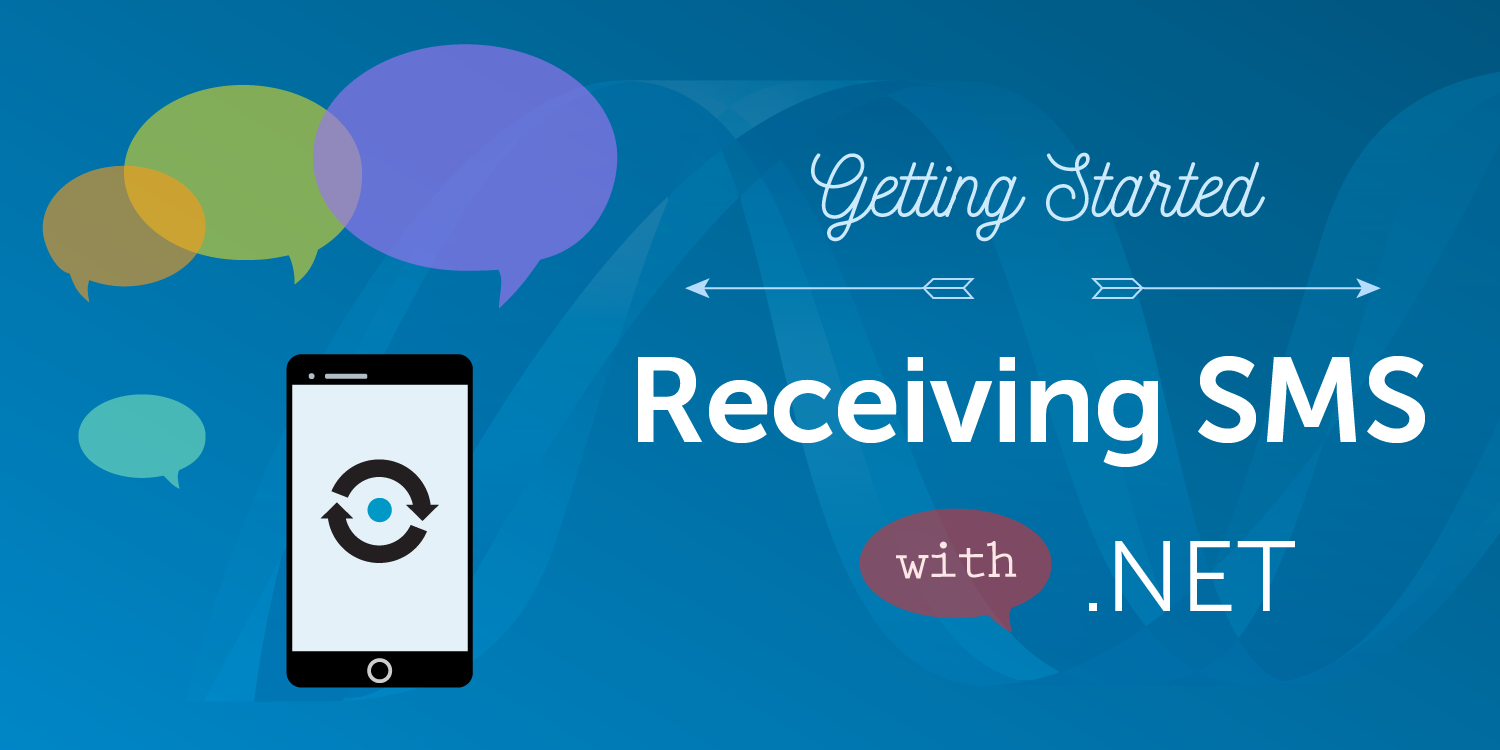
My previous tutorial explained how to use the Vonage C# Client Library to send your first SMS message from an ASP.NET MVC web app. In this follow-up, I continue exploring the Vonage Platform by showing how to receive SMS messages on a Vonage phone number using the C# Client Library.
Requirements
Visual Studio 2017 RC
Windows machine
A starter project set up in the previous blog post
Vonage API Account
To complete this tutorial, you will need a Vonage API account. If you don’t have one already, you can sign up today and start building with free credit. Once you have an account, you can find your API Key and API Secret at the top of the Vonage API Dashboard.
This tutorial also uses a virtual phone number. To purchase one, go to Numbers > Buy Numbers and search for one that meets your needs.
Receive Webhooks on Your Localhost with Ngrok
The Vonage SMS API uses webhooks to inform your ASP.NET web application that an incoming text message has been sent to one of your Vonage phone numbers. In order to do this, Vonage needs to be able to make an HTTP request to a public URL. Since we’re developing our app on our local machine, we need to create a local tunnel that gives our machine a public URL. We will be using ngrok to expose the port over the internet to easily communicate with the Vonage platform during this testing phase. This approach saves you from having to deploy your application.
Download ngrok and run the following command in Command Prompt (replace the port number with the number of the port you wish to run the app on).
Ngrok URL
The command above allows your local server (running on the port above) to have a public URL that will be used to forward the incoming texts (HTTP requests) back to your local server. The host header needs to be specified to make sure the host header of the application and ngrok match and to ensure the requests will not be rejected.
Diving Into Code
In the previous tutorial, we created an ASP.NET MVC project and added another controller called SMSController.cs
. Next, we created two action methods. One method was to present the view for the details of the SMS (destination number and message text) and the other was to retrieve the values from the form and send an SMS.
Continuing the project from the previous blog post, let's create an action method called ‘Receive’ in SMSController.cs
. This path will be receiving the inbound SMS data as we will be setting the Vonage webhook (later in this tutorial) to our ngrok URL with the route of ‘SMS/Receive’.
Add [FromUri] in the parameter to read the incoming SMS. In order to be able to use [FromUri], you need to install the following package: Microsoft.AspNet.WebApi.Core
. Above this method, add an HTTPGetAttribute to restrict the method to accepting only GET requests. If the value for response.to (the Vonage phone number) and msidsn (the sender) are not null, print out the message to the output window using Debug.WriteLine
. Else, the endpoint was hit as a result of something other than an incoming SMS. (This can happen when you first set up your webhook. We’ll see this shortly.)
[System.Web.Mvc.HttpGet]
public ActionResult Receive([FromUri]SMS.SMSInbound response)
{
if (null != response.to && null != response.msisdn)
{
Debug.WriteLine("-------------------------------------------------------------------------");
Debug.WriteLine("INCOMING TEXT");
Debug.WriteLine("From: " + response.msisdn);
Debug.WriteLine(" Message: " + response.text);
Debug.WriteLine("-------------------------------------------------------------------------");
return new HttpStatusCodeResult(200);
}
else {
Debug.WriteLine("-------------------------------------------------------------------------");
Debug.WriteLine("Endpoint was hit.");
Debug.WriteLine("-------------------------------------------------------------------------");
return new HttpStatusCodeResult(200);
}
}
Run the ASP.NET Web App
In the Solution Explorer, expand the project and click Properties. Click the Web tab and change the Project URL to the same port you are exposing via ngrok. Finally, run the project.
Project settings
Set the SMS Callback Webhook URL for Your Vonage Number
Now use the Vonage CLI to create a Vonage app and a webhook for it.
Install the Vonage CLI globally with this command:
npm install @vonage/cli -g
Next, configure the CLI with your Vonage API key and secret. You can find this information in the Developer Dashboard.
vonage config:set --apiKey=VONAGE_API_KEY --apiSecret=VONAGE_API_SECRET
Create a Voice Application
Create a new directory for your project and CD into it:
mkdir my_project
CD my_project
Now, use the CLI to create a Vonage application.
Now you need a number so you can receive calls. You can rent one by using the following command (replacing the country code with your code). For example, if you are in the USA, replace GB
with US
:
Now link the number to your app:
vonage apps:link --number=VONAGE_NUMBER APP_ID
Receive an SMS with ASP.NET
You are ready to go! With your ASP.NET web app running, open up the output window in Visual Studio. Send an SMS to your Vonage phone number and you will see the incoming texts coming through! Your ASP.NET web app is able to receive SMS messages that are sent to your Vonage phone number via an inbound webhook!
Feel free to reach out via e-mail or Twitter if you have any questions!